Angular is a powerful and versatile platform for building dynamic and scalable web applications. Whether you’re new to Angular or looking to refresh your skills, this tutorial will guide you through the process of creating your very first Angular 5 app from scratch. Angular 5, with its improved performance, enhanced tooling, and a rich set of features, has become a go-to framework for web developers who need to create fast and interactive web applications. It simplifies development through its robust structure, dependency injection, and built-in testing support, making it easier to manage complex applications.
By the end of this tutorial, you’ll not only have a functional Angular 5 app to show for your efforts but also gain a solid understanding of key Angular concepts like components, data binding, directives, and routing. This foundation will empower you to explorejs
more advanced features of Angular and tackle larger, more complex projects in the future. Ready to get started? Let’s dive into Angular 5!
What is Angular 5?
Angular 5 is a front-end web application framework developed by Google. It is an open-source, TypeScript-based framework that allows developers to build dynamic and single-page web applications. Angular 5 introduced several improvements over its predecessor, Angular 4, including better performance, a smaller bundle size, and enhanced tooling.
Before you begin, it’s important to understand the key features of Angular 5 that make it stand out:
- Improved Build Optimizations: Angular 5 comes with new tools to optimize application performance, such as the Tree-Shakable providers.
- RxJS 5.5 Support: This version of Angular integrates RxJS 5.5, which offers improved reactive programming.
- Angular CLI: The Angular Command Line Interface (CLI) simplifies the process of creating and managing Angular apps.
- Improved Universal Support: Angular 5 introduced better server-side rendering, which enhances SEO performance and reduces load time.
Now, let’s dive into building your first Angular 5 app.
Prerequisites
Before we start building the app, make sure you have the following tools installed on your machine:
- Node.js: Angular requires Node.js to run. Download and install Node.js from the official Node.js website.
Angular CLI: The Angular Command Line Interface (CLI) simplifies the process of creating Angular applications. Install it globally by running the following command in your terminal:
Bash
npm install -g @angular/cli
Step 1: Setting Up Your Angular 5 App
Once you’ve installed the necessary tools, you can create your first Angular 5 application. Here are the steps:
- Open a terminal and navigate to the folder where you want to create your project.
Run the following command to create a new Angular app:
ng new angular5-app
- You’ll be prompted to choose some configuration options:
- Would you like to add Angular routing? Type Y if you want to use routing in your app.
- Which stylesheets would you like to use? Choose CSS or another preprocessor like Sass.
3. After the setup process is completed, navigate to the project directory:
cd angular5-app
4. Finally, start the development server by running:
ng serve
You should now see the app running in your browser at http://localhost:4200.
Step 2: Exploring the Angular Project Structure
Let’s take a look at the folder structure of your newly created Angular app:
- e2e/: End-to-end testing folder for testing the Angular app.
- node_modules/: Folder containing all the dependencies installed via npm.
- src/: The source code for your app. Most of your work will happen here.
- angular.json: Configuration file for your Angular app.
- package.json: A manifest for your project containing scripts and dependencies.
The src folder is where the core of the application resides. The app directory inside src contains the main codebase for your application.
Step 3: Creating Your First Component
In Angular, components are the building blocks of the application. Let’s create a simple component to display a welcome message.
In your terminal, run the following command to generate a new component:
ng generate component welcome
- This will create the following files in the src/app directory:
- welcome.component.ts: The TypeScript file that defines the logic for the component.
- welcome.component.html: The HTML template for the component.
- welcome.component.css: The CSS for styling the component.
2. Open the welcome.component.ts file and modify the content to define a simple welcome message:
import { Component } from ‘@angular/core’;
@Component({
selector: ‘app-welcome’,
templateUrl: ‘./welcome.component.html’,
styleUrls: [‘./welcome.component.css’]
})
export class WelcomeComponent {
message: string = ‘Welcome to Angular 5!’;
}
3. Open the welcome.component.html file and add the following HTML code:
<div>
<h1>{{ message }}</h1>
</div>
Step 4: Displaying the Component in the App
Now that we’ve created the WelcomeComponent, let’s display it in the main app.component.html file.
Open src/app/app.component.html and replace its contents with the following:
<app-welcome></app-welcome>
This tells Angular to use the WelcomeComponent inside the main component.
Step 5: Running the Angular App
Now that you’ve created and linked your first component, it’s time to see your app in action:
If you haven’t already, start the Angular development server:
ng serve
- Open your browser and navigate to http://localhost:4200. You should see the welcome message displayed in your app.
Step 6: Understanding Angular Directives and Data Binding
Angular 5 offers powerful features like directives and data binding. In this step, we’ll briefly explain both concepts and how they’re used.
Data Binding: Angular uses data binding to keep the view and model in sync. There are several types of data binding in Angular:
Interpolation: You’ve already seen interpolation in action in the welcome.component.html file:
<h1>{{ message }}</h1>
- This binds the message property in the component class to the view.
Property Binding: You can bind properties like HTML attributes to component properties:
<img [src]=”imageUrl” />
Directives: Directives are special markers on elements that add behavior to them. There are two types of directives:
- Structural Directives: These change the structure of the DOM (e.g., *ngIf, *ngFor).
- Attribute Directives: These change the appearance or behavior of an element (e.g., ngClass, ngStyle).
Step 7: Adding Routing to Your Angular App
To add routing to your Angular 5 app, follow these steps:
In the app.module.ts file, import RouterModule and Routes:
import { RouterModule, Routes } from ‘@angular/router’;
Define your routes:
const routes: Routes = [
{ path: ”, component: WelcomeComponent }
];
Add the RouterModule to the imports array of @NgModule:
@NgModule({
declarations: [AppComponent, WelcomeComponent],
imports: [RouterModule.forRoot(routes)],
bootstrap: [AppComponent]
})
In the app.component.html file, add the <router-outlet></router-outlet> directive where you want the routed content to appear.
Conclusion
Congratulations! You’ve successfully built your first Angular 5 app. In this tutorial, you learned the essential steps to get started with Angular, including setting up your environment, creating a component, integrating it into your app, and mastering key Angular concepts such as data binding, directives, and routing. These fundamental skills lay the groundwork for developing more complex and interactive web applications.
To take your skills further, you can dive into more advanced topics like Angular services, forms, and state management, which will help you build scalable and maintainable apps. The Angular framework offers robust tools for optimizing performance and ensuring smooth user experiences across a variety of devices.
For more in-depth knowledge, explore these valuable resources:
By continuing to explore and experiment with Angular 5’s rich features, you’ll become a proficient Angular developer, equipped to tackle sophisticated development challenges with ease.
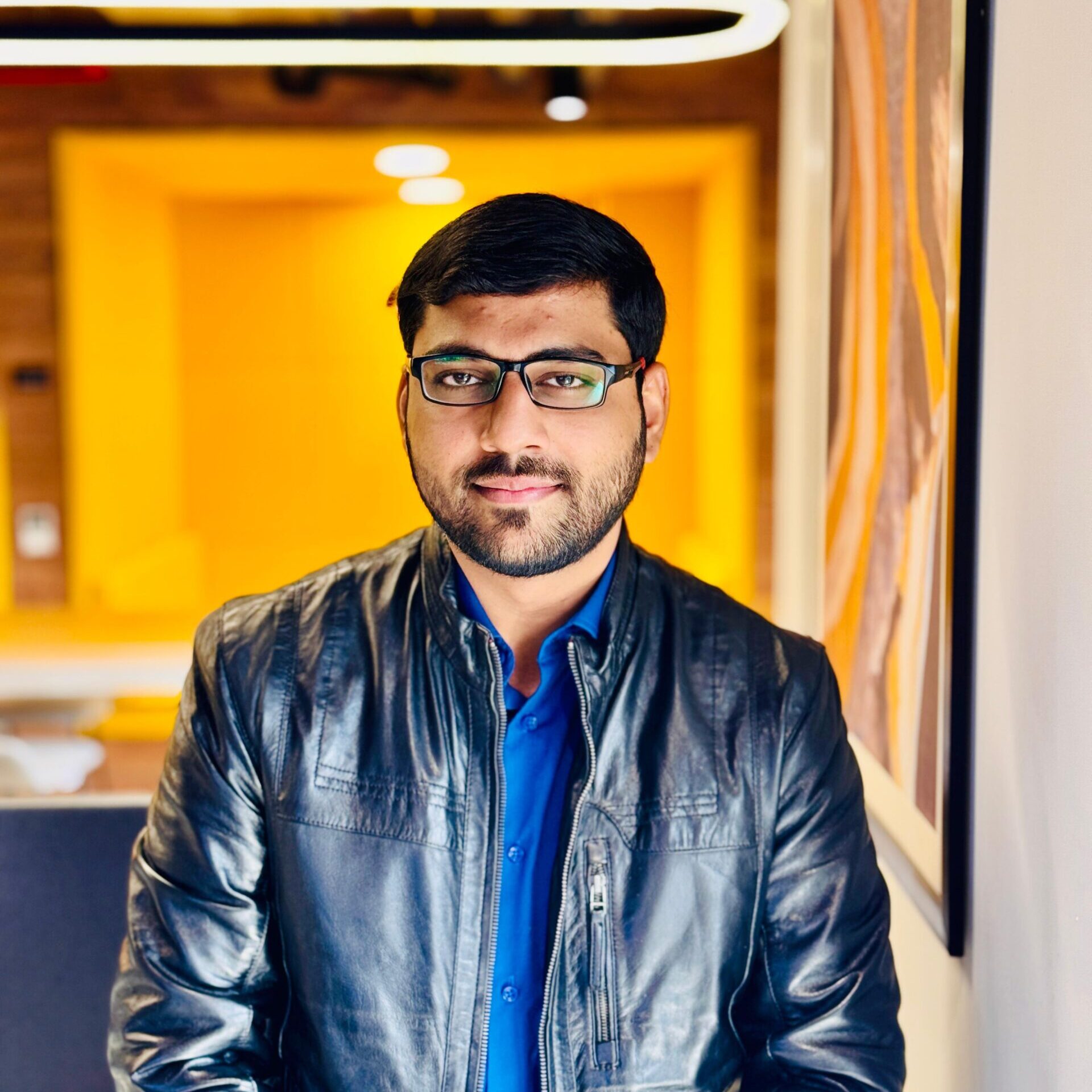
Jahanzaib is a Content Contributor at Technado, specializing in cybersecurity. With expertise in identifying vulnerabilities and developing robust solutions, he delivers valuable insights into securing the digital landscape.
One Response
Your article helped me a lot, is there any more related content? Thanks!